C programs are done on a terminal that is not stored anywhere. In the software industry, most programs are written to store the information fetched from the program. One such way is to store the fetched information in a file.
Why files are needed?
If you have to enter a large number of data, it will take a lot of time to enter them all.
However, if you have a file containing all the data, you can easily access the contents of the file using a few commands in C and you can easily move your data from one computer to another without any changes.
File Types :
- Text files
- Binary files
1. Text files:
Text files are the normal .txt files. You can easily create text files using any simple text editor such as Notepad. When you open text files, you’ll see all the contents within the file as plain text. It takes minimum effort to maintain, are easily readable, and provides the least security, and takes bigger storage space.
2. Binary files:
Binary files are mostly the .bin files on your computer. Instead of storing data in plain text, they store it in the binary form. They can hold a higher amount of data, are not readable easily, and provides better security than text files.
Operations of Files:
- Creating a new file
- Opening an existing file
- Closing a file
- Reading from and writing information to a file
When working with files, you need to declare a pointer of type file.
FILE *fptr;
Opening a file – for creation and edit:
A file opening is performed using the fopen()
function defined in the stdio.h
header file.
ptr = fopen("fileopen","mode");
Opening Modes in Standard I/O
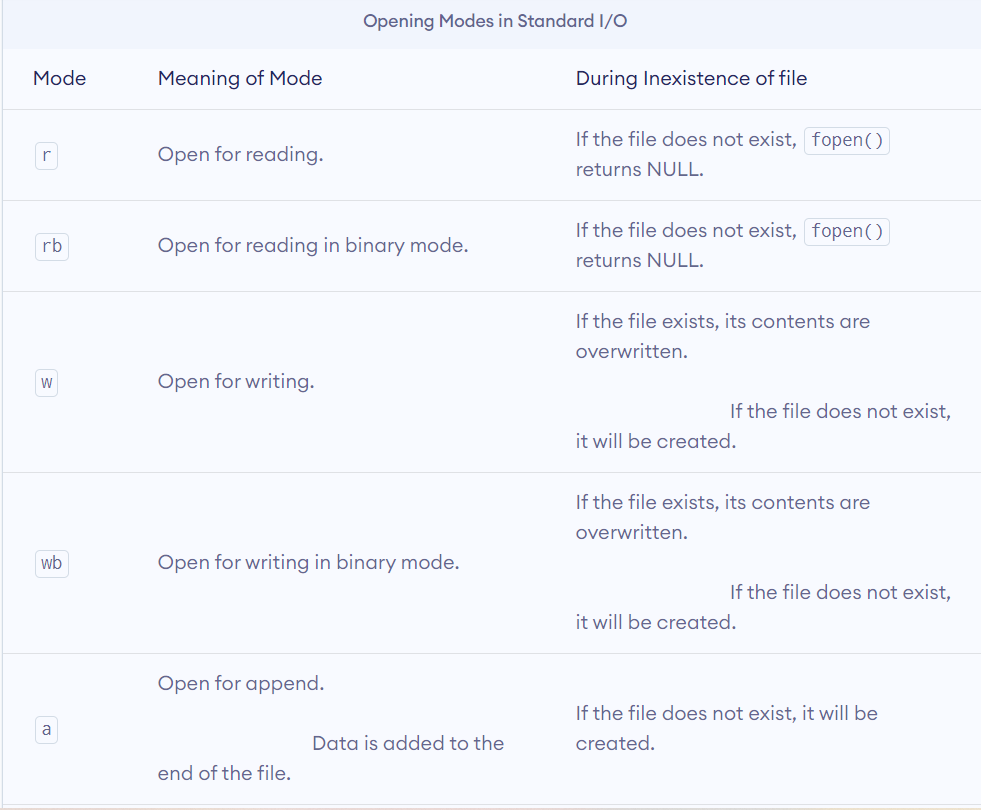
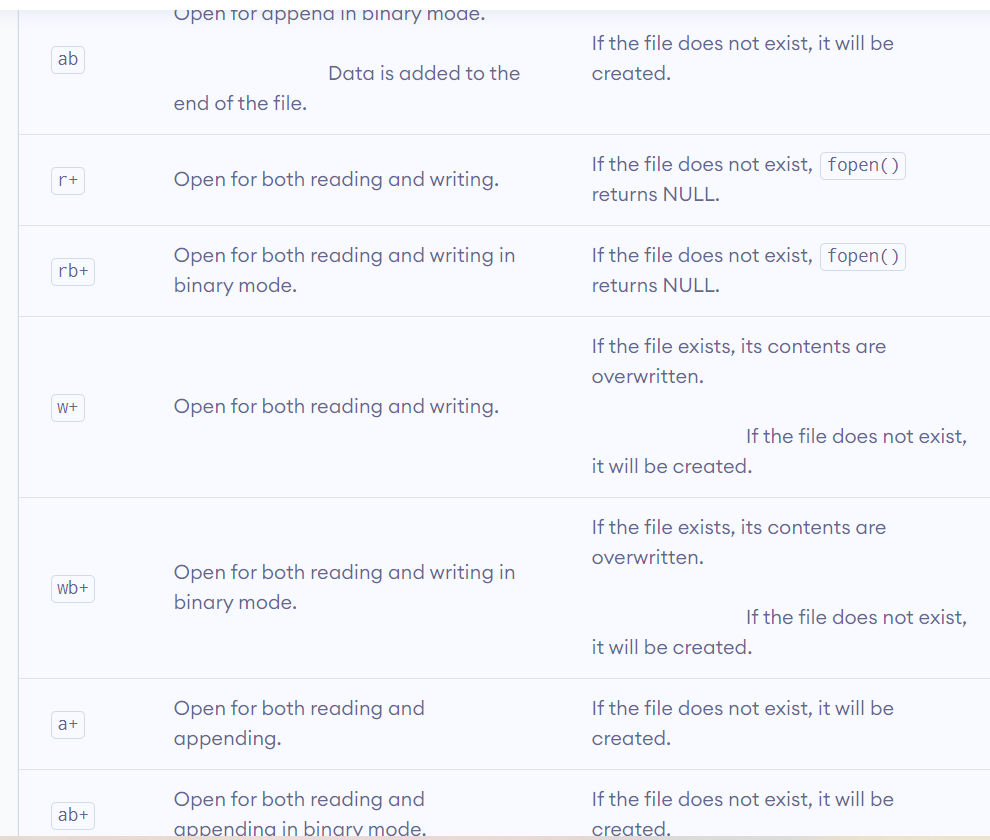
Closing a file :
Closing a file is performed using the fclose()
function.
fclose(fptr);
Here, fptr
is a file pointer associated with the file to be closed.
Example : Program to Open a File, Write in it, And Close the File
// C program to Open a File,
// Write in it, And Close the File
# include <stdio.h>
# include <string.h>
int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Get the data to be written in file
char dataToBeWritten[50]
= "GeeksforGeeks-A Computer Science Portal for Geeks";
// Open the existing file GfgTest.c using fopen()
// in write mode using "w" attribute
filePointer = fopen("GfgTest.c", "w") ;
// Check if this filePointer is null
// which maybe if the file does not exist
if ( filePointer == NULL )
{
printf( "GfgTest.c file failed to open." ) ;
}
else
{
printf("The file is now opened.\n") ;
// Write the dataToBeWritten into the file
if ( strlen ( dataToBeWritten ) > 0 )
{
// writing in the file using fputs()
fputs(dataToBeWritten, filePointer) ;
fputs("\n", filePointer) ;
}
// Closing the file using fclose()
fclose(filePointer) ;
printf("Data successfully written in file GfgTest.c\n");
printf("The file is now closed.") ;
}
return 0;
}
Example : Program to Open a File, Read from it, And Close the File
// C program to Open a File,
// Read from it, And Close the File
# include <stdio.h>
# include <string.h>
int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Declare the variable for the data to be read from file
char dataToBeRead[50];
// Open the existing file GfgTest.c using fopen()
// in read mode using "r" attribute
filePointer = fopen("GfgTest.c", "r") ;
// Check if this filePointer is null
// which maybe if the file does not exist
if ( filePointer == NULL )
{
printf( "GfgTest.c file failed to open." ) ;
}
else
{
printf("The file is now opened.\n") ;
// Read the dataToBeRead from the file
// using fgets() method
while( fgets ( dataToBeRead, 50, filePointer ) != NULL )
{
// Print the dataToBeRead
printf( "%s" , dataToBeRead ) ;
}
// Closing the file using fclose()
fclose(filePointer) ;
printf("Data successfully read from file GfgTest.c\n");
printf("The file is now closed.") ;
}
return 0;
}
Example : Write to a text file
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
FILE *fptr;
// use appropriate location if you are using MacOS or Linux
fptr = fopen("C:\\program.txt","w");
if(fptr == NULL)
{
printf("Error!");
exit(1);
}
printf("Enter num: ");
scanf("%d",&num);
fprintf(fptr,"%d",num);
fclose(fptr);
return 0;
}
This program takes a number from the user and stores in the file program.txt
. When you open the file, you can see the integer you entered.
Example : Read from a text file
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
FILE *fptr;
if ((fptr = fopen("C:\\program.txt","r")) == NULL){
printf("Error! opening file");
// Program exits if the file pointer returns NULL.
exit(1);
}
fscanf(fptr,"%d", &num);
printf("Value of n=%d", num);
fclose(fptr);
return 0;
}
This program reads the integer present in the program.txt
file and prints it onto the screen. Other functions like fgetchar()
, fputc()
etc. can be used in a similar way.
Example : Write to a binary file using fwrite()
#include <stdio.h>
#include <stdlib.h>
struct threeNum
{
int n1, n2, n3;
};
int main()
{
int n;
struct threeNum num;
FILE *fptr;
if ((fptr = fopen("C:\\program.bin","wb")) == NULL){
printf("Error! opening file");
// Program exits if the file pointer returns NULL.
exit(1);
}
for(n = 1; n < 5; ++n)
{
num.n1 = n;
num.n2 = 5*n;
num.n3 = 5*n + 1;
fwrite(&num, sizeof(struct threeNum), 1, fptr);
}
fclose(fptr);
return 0;
}
Example : Read from a binary file using fread()
#include <stdio.h>
#include <stdlib.h>
struct threeNum
{
int n1, n2, n3;
};
int main()
{
int n;
struct threeNum num;
FILE *fptr;
if ((fptr = fopen("C:\\program.bin","rb")) == NULL){
printf("Error! opening file");
// Program exits if the file pointer returns NULL.
exit(1);
}
for(n = 1; n < 5; ++n)
{
fread(&num, sizeof(struct threeNum), 1, fptr);
printf("n1: %d\tn2: %d\tn3: %d", num.n1, num.n2, num.n3);
}
fclose(fptr);
return 0;
}
In this program, you read the same file program.bin
and loop through the records one by one. In simple terms, you read one threeNum
record of threeNum
size from the file pointed by *fptr into the structure num.
Getting data using fseek()
If you have many records inside a file and need to access a record at a specific position, you need to loop through all the records before it to get the record. An easier way to get to the required data can be achieved using fseek()
.
fseek(FILE * stream, long int offset, int whence);

Example : fseek()
#include <stdio.h>
#include <stdlib.h>
struct threeNum
{
int n1, n2, n3;
};
int main()
{
int n;
struct threeNum num;
FILE *fptr;
if ((fptr = fopen("C:\\program.bin","rb")) == NULL){
printf("Error! opening file");
// Program exits if the file pointer returns NULL.
exit(1);
}
// Moves the cursor to the end of the file
fseek(fptr, -sizeof(struct threeNum), SEEK_END);
for(n = 1; n < 5; ++n)
{
fread(&num, sizeof(struct threeNum), 1, fptr);
printf("n1: %d\tn2: %d\tn3: %d\n", num.n1, num.n2, num.n3);
fseek(fptr, -2*sizeof(struct threeNum), SEEK_CUR);
}
fclose(fptr);
return 0;
}
This program will start reading the records from the file program.bin
in the reverse order and prints it.